Extending the GitHub CLI
I know I've mentioned the GitHub command line tool, gh.exe
, in the past. This is a great tool for working with GitHub repositories from the command line. I use it all the time and have built some automation around it. You can install it with winget or other package managers.
winget Install github.cli
Or you can download directly from https://cli.github.com/. This is also where you can find the documentation.
I bring this up because the other day I discovered a new feature of gh.exe
that I didn't know about. You can extend the functionality of gh.exe
with extensions. You can think of an extension as a plug-in. The extension does just what the name implies, it extends the functionality of gh.exe
.
Extensions are written in a number of languages like Go, Rust, and Python. The extensions use the GitHub API to achieve an end result. You don't have to worry about the language the extension is written in. You just install the extension that meets your need and use it.
Finding Extensions
You can use gh.exe
to search for extensions.
PS C:\> gh extension search --help
Usage: gh extension search [<query>] [flags]
Flags:
-q, --jq expression Filter JSON output using a jq expression
--json fields Output JSON with the specified fields
--license strings Filter based on license type
-L, --limit int Maximum number of extensions to fetch (default 30)
--order string Order of repositories returned, ignored unless '--sort' flag is specified: {asc|desc} (default "desc")
--owner strings Filter on owner
--sort string Sort fetched repositories: {forks|help-wanted-issues|stars|updated} (default "best-match")
-t, --template string Format JSON output using a Go template; see "gh help formatting"
-w, --web Open the search query in the web browser
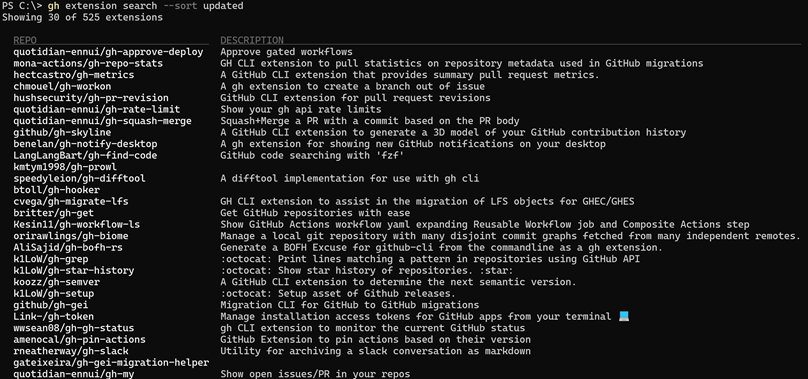
Or maybe you want to search for a topic or key word.
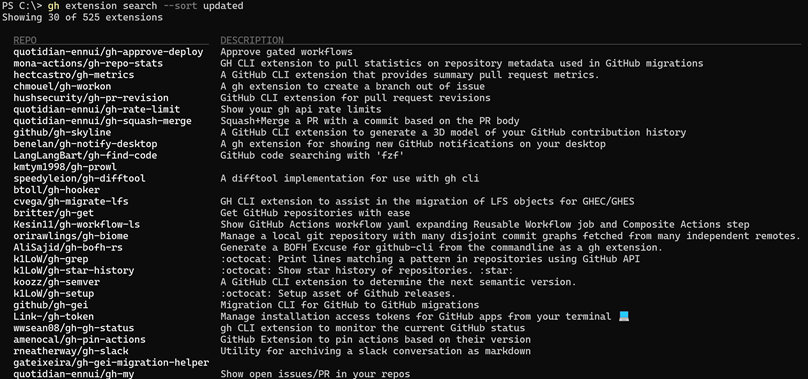
Some of the gh.exe
parameters provide a formatting option which allows you to the output as JSON. When it comes to searching extensions you can use any of these JSON field.
createdAt
defaultBranch
description
forksCount
fullName
hasDownloads
hasIssues
hasPages
hasProjects
hasWiki
homepage
id
isArchived
isDisabled
isFork
isPrivate
language
license
name
openIssuesCount
owner
pushedAt
size
stargazersCount
updatedAt
url
visibility
watchersCount
The field name is case-sensitive, and specify them as a comma-separated list.
PS C:\> gh extension search branch --sort updated --json fullName,url,updatedAt,description --limit 1
[
{
"description": "A gh extension to create a branch out of issue",
"fullName": "chmouel/gh-workon",
"updatedAt": "2024-12-16T09:25:39Z",
"url": "https://github.com/chmouel/gh-workon"
}
]
Of course, once you have JSON, you can create objects you can work with in PowerShell.
gh extension search branch --sort updated --json fullName,url,updatedAt,description |
ConvertFrom-Json |
ForEach-Object {
[PSCustomObject]@{
PSTypeName = 'ghExtensionSearchResult'
Name = $_.fullName
Updated = $_.updatedAt
Url = $_.url
Description = $_.description
}
} | Sort Updated -Descending
> ⚠ Warning > If the description includes emojis, the emoji won't be displayed properly
It wouldn't take much to turn this into a function that you could use to search for extensions.
Even though I'm sorting in the initial gh.exe
command, the sort doesn't appear to be correct so I am sorting again after I create the custom object. I'm creating a custom object so that I can define a typename and provide more meaningful property names.

If you prefer, you can open the search results in a browser.
gh extension search -w
This will open a page on GitHub.com additional filtering options. But my favorite way to search is to use the browse option.
gh extension browse
This will open a terminal user interface (TUI) where you can browse and search for extensions.
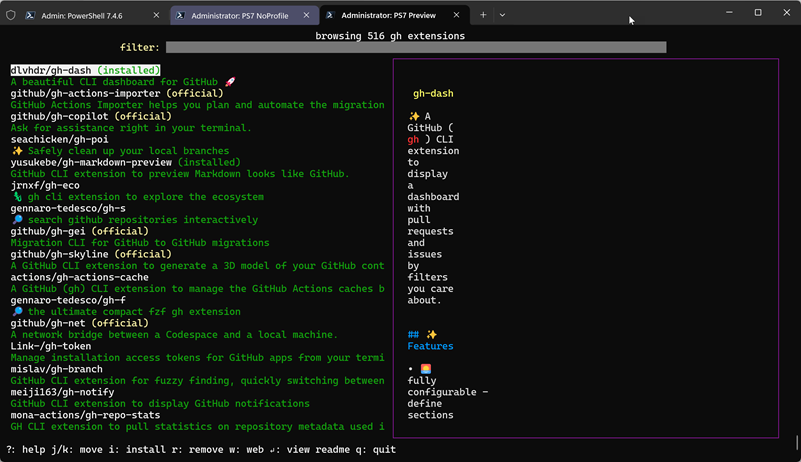
You can use j
and k
to move up and down the list or the arrow keys. The extension's README file is displayed on the right. If you find an extension you want to know more about, press w
which will open the extension's GitHub project page in your browser. This is a great way to view the complete README file and any other information the author has provided.
If you want to filter, press /
and type a search term in the filter box.
When you are finished browsing, press q
to quit.
Installing Extensions
If you are browsing, you can easily install an extension by pressing i
. Otherwise, install the extension with the full name
gh extension install joaom00/gh-b
If you are building PowerShell tools, you need to specify the extension using the
format.