Super Size Your Snippets
Today I want to return to a topic I've covered, at least briefly, in the past and that is taking advantage of snippets in Visual Studio Code. The PowerShell ISE also supports snippets, but since that tool is considered deprecated and is limited to PowerShell, I'm not going to cover that tool today. VS Code is a much more versatile tool and is my preferred editor for PowerShell scripting, writing Markdown documents, working with JSON files, and much more. Each of these tasks requires a different file type and VS Code allows me to create and leverage snippets for each file type. All of this makes me more efficient.
VS Code ships with many built-in snippets for PowerShell, but you can create your own. You can also create snippets for other file types. In this article, I'll show you how to create and use snippets in VS Code.
The Snippet Manager
UPDATE A reader alerted me after I published this that the Snippet Manager is part of the Easy Snippet VS Code extension which I mention later in the article. You will want the extension anyway so you'll need to install it for the rest of this article to make any sense. Sorry for the confusion.
The first thing to do is to open the Snippet Manager. In the left sidebar, also known as the Activity Bar, you can click the snippets icon.
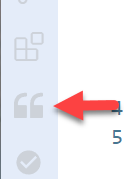
If the Activity Bar is hidden, open the Command Palette with Ctrl+Shift+P
and start typing activity
.
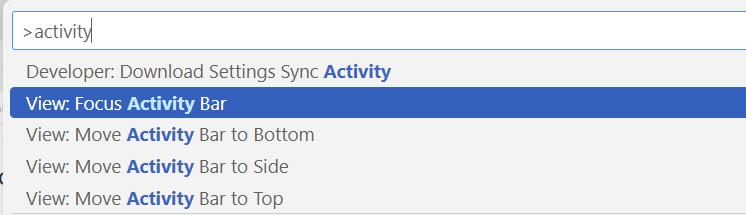
Select Focus Activity Bar
to restore it.
When you click on the snippets icon, you'll open the Snippet Manager.
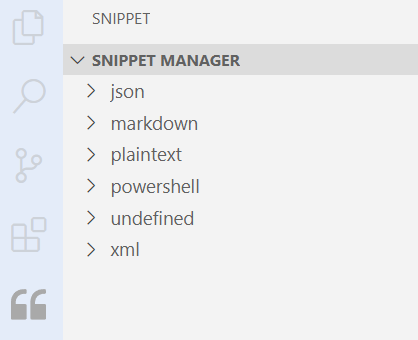
Click on one the languages to expand it.
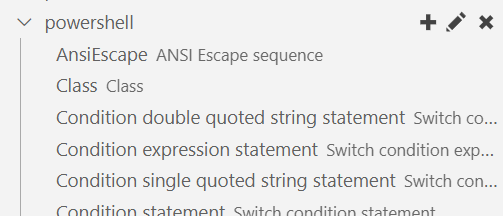
Inserting Snippets
In the snippet list, you'll see the snippet name followed by a brief description. I'll move to a PowerShell file and start type ansi
. VSCode will begin to auto-complete the snippet name. I can press Ctrl+Space
to see snippet detail.
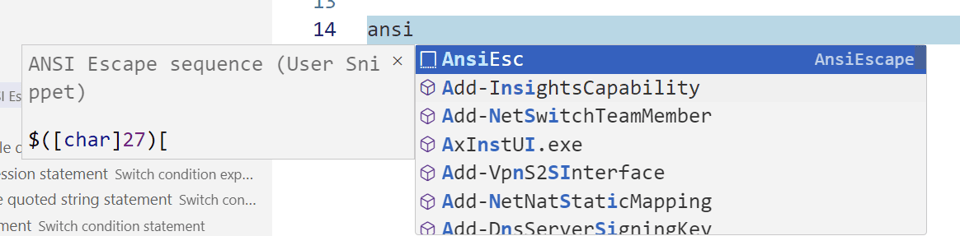
Pressing Enter
will insert the snippet.
$([char]27)[
This is a simple example.
Some of the PowerShell snippets have placeholders. I'll insert the If
snippet into my PowerShell script file.
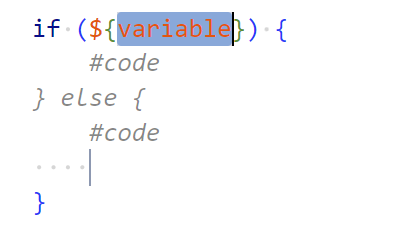
Type your PowerShell code in the selected area.
if (${$x -gt 10 }) {
}
I have to admit I don't like the way some of these snippets are setup with the extra braces. I typically will revised the inserted code.
if ($x -gt 10 ) {
}
You will also encounter snippets like this. I've inserted the for
snippet into my PowerShell script.
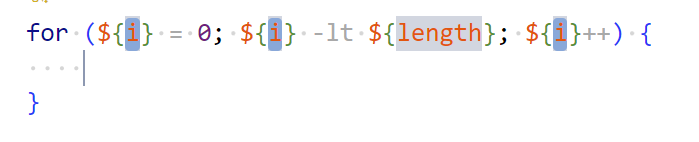
You can see the highlighted elements. Notice the placeholder i
is selected and shown three times. I can type x
as my variable and it is changed in all three places.

I can press Tab
to move to the next placeholder and insert a value.
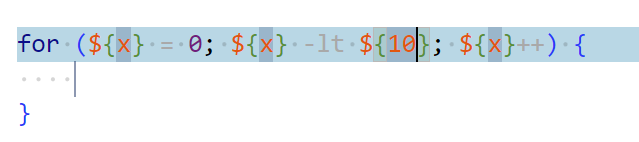
This leaves me with this code in my file.
for (${x} = 0; ${x} -lt ${10}; ${x}++) {
}
Which I'll revise.
for ($x = 0; $x -lt 10; $x++) {
}
I'll revisit placeholders later.
Creating Snippets
While the built-in snippets are handy, I expect you have bits of code you re-use all the time. Turn them into a snippet. I'm going to create a new PowerShell snippet that will insert the ANSI reset sequence.
In the Snippet Manager, click the +
icon next to the appropriate language. Insert the key for your snippet.

This is the value you will type in the VSCode editor to insert the snippet. VSCode will open a new tab where you can define the snippet.