Native PSSession PowerShell Scripting
The exploration of CIM and using the `CimSession` .NET class in PowerShell scripting got me thinking about another remoting option, `PSSession`. This could be another option for creating PowerShell scripts with remoting baked in. I'd like to have a function that doesn't rely on parameter sets to distinguish between a computer name and an existing PSSession.
However, unlike `CimSession`, I can't easily create a `PSSession` object from a computer name. There is a little more involved, but it isn't too difficult. I thought I'd demonstrate how to crate and use a `PSSession` object from scratch. If nothing else, hopefully, you'll learn a little more about how PowerShell remoting works under the hood and maybe pickup a scripting idea or two.
What I want to demonstrate requires PowerShell 7. I'm going to rely on a static method that doesn't exist in Windows PowerShell. But remember, you can still remotely connect to and manage systems running Windows PowerShell from a PowerShell 7 desktop. I am also leveraging traditional PowerShell remoting which means a Windows platform. Although I will show you how to setup an SSH PowerShell remoting session from scratch.
There are several pieces to this puzzle. Creating a `PSSession` object is not as easy as what I showed you with `CimSession`. We know we ultimately need to create a `System.Management.Automation.Runspaces.PSSession` object. It was easy enough to create a session using `New-PSSession` and getting the type name with `Get-Member`. I'll dig deeper using [`Get-TypeMember`](https://bit.ly/3JVpGLB).
PS C:\> Get-TypeMember System.Management.Automation.Runspaces.PSSession -Name Create | Select -ExpandProperty Syntax
$obj.Create([Runspace]runspace,[String]transportName,[PSCmdlet]psCmdlet)
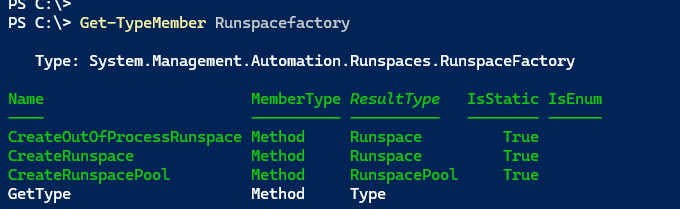
PS C:\> Get-TypeMember RunspaceFactory -Name CreateRunspace | Select-Object -ExpandProperty Syntax
$obj.CreateRunspace()
$obj.CreateRunspace([PSHost]host)
$obj.CreateRunspace([InitialSessionState]initialSessionState)
$obj.CreateRunspace([PSHost]host,[InitialSessionState]initialSessionState)
$obj.CreateRunspace([RunspaceConnectionInfo]connectionInfo,[PSHost]host,[TypeTable]typeTable)
$obj.CreateRunspace([RunspaceConnectionInfo]connectionInfo,[PSHost]host,[TypeTable]typeTable,[PSPrimitiveDictionary]applicationArguments)
$obj.CreateRunspace([RunspaceConnectionInfo]connectionInfo,[PSHost]host,[TypeTable]typeTable,[PSPrimitiveDictionary]applicationArguments,[String]name)
$obj.CreateRunspace([PSHost]host,[RunspaceConnectionInfo]connectionInfo)
$obj.CreateRunspace([RunspaceConnectionInfo]connectionInfo)
PS C:\>[System.Management.Automation.Runspaces.
The exploration of CIM and using the CimSession
.NET class in PowerShell scripting got me thinking about another remoting option, PSSession
. This could be another option for creating PowerShell scripts with remoting baked in. I'd like to have a function that doesn't rely on parameter sets to distinguish between a computer name and an existing PSSession.
However, unlike CimSession
, I can't easily create a PSSession
object from a computer name. There is a little more involved, but it isn't too difficult. I thought I'd demonstrate how to crate and use a PSSession
object from scratch. If nothing else, hopefully, you'll learn a little more about how PowerShell remoting works under the hood and maybe pickup a scripting idea or two.
What I want to demonstrate requires PowerShell 7. I'm going to rely on a static method that doesn't exist in Windows PowerShell. But remember, you can still remotely connect to and manage systems running Windows PowerShell from a PowerShell 7 desktop. I am also leveraging traditional PowerShell remoting which means a Windows platform. Although I will show you how to setup an SSH PowerShell remoting session from scratch.