Master Your Environment
PowerShell, as its name implies, is a shell. It is an interactive command-line interface to the underlying operating system. PowerShell is designed so that you can interact with the operating system, often at a very low level. This is possible because PowerShell is built on top of the .NET Framework, which provides a rich set of APIs for interacting with the operating system. You don't have to be a .NET developer to use PowerShell, but it helps to understand how the .NET Framework works.
Today, I want to talk about your environment, specifically the operating system environment. In the .NET Framework this is exposed through the System.Environment
class. PowerShell abstracts much of this class in the $Env:
PSDrive and the Environment
provider. However, there are some features of the System.Environment
class that are not explicitly exposed in PowerShell. But you can still access them using the System.Environment
class directly.
I'm sure I've shown you some of today's content before, but a refresher never hurts. And I'm sure there are some things I haven't shown you yet. So let's dive in.
[System.Environment]
The System.Environment
class is a static class that provides information about, and control over, the current environment. It provides properties and methods for working with the operating system environment, such as the current directory, the command-line arguments, and the environment variables. It is a static class, meaning you don't create an instance of it like you would a process or service object. The class simply is.
Because it is a static class, it isn't as easy to use Get-Member
to discover its properties and methods. That's why I wrote the Get-TypeMember
function which is part of the PSScriptTools module. Specify the .NET class name and the function will return a list of properties and methods. Here's how you can use it to explore the System.Environment
class:
Get-TypeMember System.Environment -MemberType Property
In PowerShell, the System
namespace is available so you can omit it from .NET class names.
Get-TypeMember Environment -MemberType Property
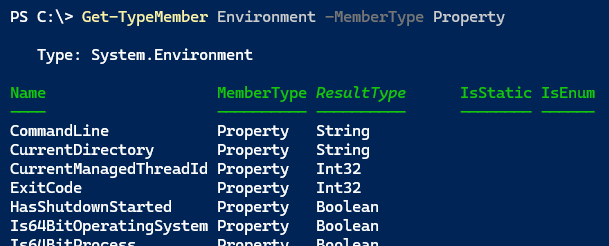
Let's look at some of these properties in more detail.
Environment Properties
You should be able to find most of these properties also defined in the $Env:
PSDrive.
PS C:\> $env:USERNAME
Jeff
PS C:\> $env:Computername
PROSPERO
Although the names might vary. Using the Get-TypeMember
command, here's a code snippet that shows you the native properties and their values. I'm also showing the syntax you would use.
Get-TypeMember System.Environment -MemberType Property |
Select-Object Name,
@{Name="Syntax";Expression={"[Environment]::{0} " -f $_.name }},
@{Name="Value";Expression = {[system.environment]::$($_.name) }}
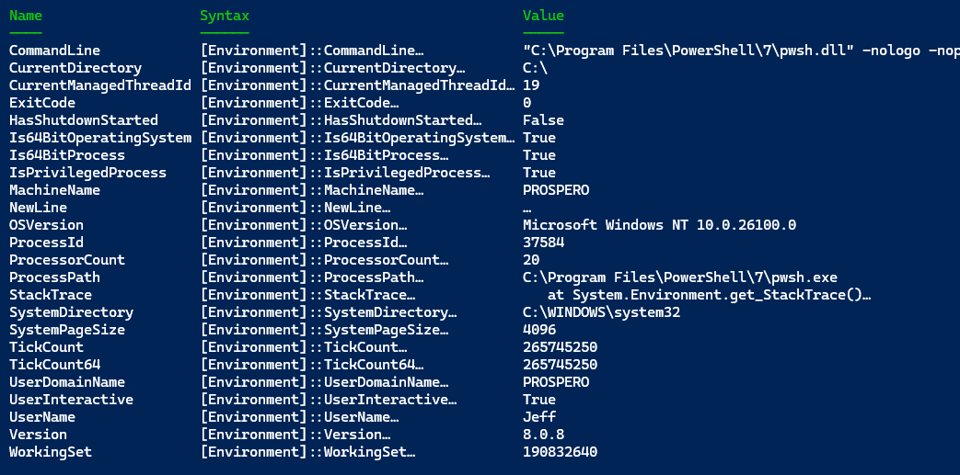
A few of these properties might be of interest to you, especially when they aren't exposed in the $Env:
PSDrive. The CommandLine
property will show you what command started the current session.
PS C:\> [Environment]::CommandLine
powershell.exe -nologo -noexit -noprofile -file c:\scripts\miniprofile51.ps1
This will vary by operating system and PowerShell version.
PS /home/jeff> [environment]::CommandLine
/opt/microsoft/powershell/7/pwsh.dll
You could also check the ProcessPath
property on PowerShell 7
PS C:\> [environment]::ProcessPath
C:\Program Files\PowerShell\7\pwsh.exe
This property is not available in Windows PowerShell.
The Version
property is the version number of the .NET Framework that PowerShell is using.
PS /home/jeff> [environment]::Version
Major Minor Build Revision
----- ----- ----- --------
8 0 4 -1
This can be a handy way of checking the version if you are using code that might be dependant on a specific version of the .NET Framework. In Windows PowerShell, this information is also stored in the $PSVersionTable
automatic variable.
PS C:\> [Environment]::Version
Major Minor Build Revision
----- ----- ----- --------
4 0 30319 42000
PS C:\> $PSVersionTable.CLRVersion
Major Minor Build Revision
----- ----- ----- --------
4 0 30319 42000
This $PSVersionTable
property is not available in PowerShell 7.
The MachineName
property is especially useful when working cross-platform because there is no $env:Computername
setting.