Location Tricks with PowerShell
I'm sure it comes as no surprise to you that I spend a lot of time at a PowerShell prompt. I am always looking for ways to minimize the amount of typing I have to do and to be as efficient as possible. Lately, I've been thinking about locations in PowerShell and how to get the most out of them. I have a few ideas I want to share with you.
Provider Aware Prompt
My first tip is a customized prompt function that is provider-aware. This function will display the current location in an associated color style based on the underlying provider.
PS C:\work> Get-Location | Format-List *
Drive : C
Provider : Microsoft.PowerShell.Core\FileSystem
ProviderPath : C:\work
Path : C:\work
PS C:\work> cd hklm:\SOFTWARE\Microsoft\
PS HKLM:\SOFTWARE\Microsoft\> Get-Location | Select-Object Provider
Provider
--------
Microsoft.PowerShell.Core\Registry
My prompt will display the current location in a different color depending on the provider. I'm also testing if I am running in an elevated session on Windows.
$AdminFG = "Gray"
If ($IsWindows -OR ($PSEdition -eq 'desktop')) {
$user = [Security.Principal.WindowsIdentity]::GetCurrent()
If ( (New-Object Security.Principal.WindowsPrincipal $user).IsInRole([Security.Principal.WindowsBuiltinRole]::Administrator)) {
$AdminFG = 'Red'
}
}
I set an initial value for $AdminFG
. On Windows, I could have used the default foreground color, $host.UI.RawUI.ForegroundColor
. However, on non-Windows systems, this isn't defined so I'll explicitly set a console color value.
My sample prompt is a variation on the default prompt function.
Function prompt {
# .Description
# This custom version of the PowerShell prompt will present a colorized location value based on the current provider. It will also display the PS prefix in red if the current user is running as administrator.
# .Link
# https://go.microsoft.com/fwlink/?LinkID=225750
# .ExternalHelp System.Management.Automation.dll-help.xml
#Non-Windows PowerShell hosts don't have a ForegroundColor defined
$AdminFG = "Gray" #or use default $host.UI.RawUI.ForegroundColor
If ($IsWindows -OR ($PSEdition -eq 'desktop')) {
$user = [Security.Principal.WindowsIdentity]::GetCurrent()
If ( (New-Object Security.Principal.WindowsPrincipal $user).IsInRole([Security.Principal.WindowsBuiltinRole]::Administrator)) {
$AdminFG = 'Red'
}
}
#As an alternative you could use $PSStyle
Switch ((Get-Location).provider.name) {
'FileSystem' { $fg = 'green' }
'Registry' { $fg = 'magenta' }
'wsman' { $fg = 'cyan' }
'Environment' { $fg = 'yellow' }
'Certificate' { $fg = 'DarkCyan' }
'Function' { $fg = 'blue' }
'Alias' { $fg = 'DarkGray' }
'Variable' { $fg = 'DarkGreen' }
Default { $fg = 'Gray' }
}
Write-Host "[$(Get-Date -Format 'hh\:mm\:ss\.ffff')] " -NoNewline
Write-Host 'PS ' -NoNewline -ForegroundColor $AdminFG
Write-Host "$($executionContext.SessionState.Path.CurrentLocation.Path)" -ForegroundColor $fg -NoNewline
Write-Output "$('>' * ($nestedPromptLevel + 1)) "
}
This function will display the current location in a color that corresponds to the provider. It will also display the PS
prefix in red if the current user is running as an administrator.
Figure 1 shows a sample of the prompt in action:
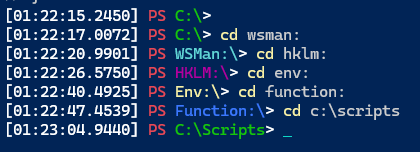
This won't rock your world, but it might be nice to have a little cue at your prompt. If you have an existing prompt function, you might want to incorporate some of my ideas.
The other location-related topic I'm working on is related to jump lists. That is, making it even easier to jump to previous locations.