Creating PowerShell Custom Formatting
We all know the importance of objects in PowerShell. PowerShell works with objects and lets you manipulate them in the pipeline. At the end of the process, PowerShell formats and displays whatever remains in the pipeline using the default formatting rules. You can control the output by using formatting cmdlets like Format-Table
or Format-List
. These commands rely on formatting definitions. In Windows PowerShell, these are stored as ps1xml files in $PSHome
. In PowerShell 7, these files are stored internally for better performance.
The formatting files will define how the objects are displayed.
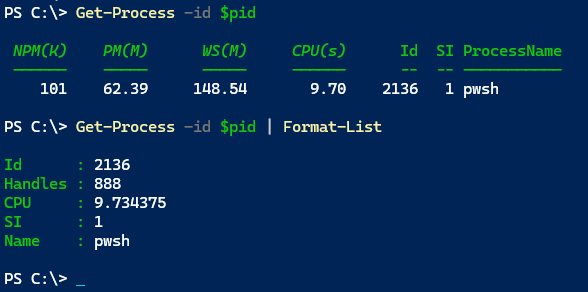
You can create formatting files for your custom objects. Typically, we will create table and list views. You'll find using my New-PSFormatXml
command from the PSScriptTools module will make this process easier.
However, there is another formatting option that is often overlooked. It is not well documented and you'll need to spend some time experimenting to get it right, but it might be worth the effort. This is creating custom formatting. Instead of piping your object to Format-Table
or Format-List
, you will use Format-Custom
. You will still need a ps1xml file, which I'll get to. If this is the only file for your custom object, PowerShell will default to using it. Or you might want to create custom views for other object types in PowerShell.
To begin with, you will need an XML template.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<viewdefinitions>
<view>
<name>VIEW-NAME</name>
<viewselectedby>
<typename>OBJECT-TYPENAME</typename>
</viewselectedby>
<customcontrol>
<customentries>
<customentry>
<customitem>
<!-- ################ CUSTOM DEFINITIONS ################ -->
<!-- the template needs at least one item-->
<newline></newline>
</customitem>
</customentry>
</customentries>
</customcontrol>
</view>
</viewdefinitions>
</configuration>
> Don't forget that XML tags are case-sensitive. This has burned me more than once.
You will want to give your view a name. You can use the object type name or something else. Remember, you need a unique type name. This will not work for a PSCustomObject
type.
<viewdefinitions>
<view>
<name>default</name>
<viewselectedby>
<typename>PSFooBarThing</typename>
</viewselectedby>
It is possible to have multiple views for the same object type in the same file. Make sure you give each view a unique name.
Custom Item
You will define a custom item formatting that will be applied to every object. I'll start with something simple. Keep in mind that everything you want to display will be treated as a string.
Within your custom item, you can, in fact, write a static string.
<customitem>
<text>##################</text>
All output is sequential, but you can insert blank lines.
<customitem>
<text>##################</text>
<newline></newline>