“Don't criticize what you can't understand.” - Bob Dylan
Hey there!
Last week, if you remember, I had posted a photo of a recent trip I took in the newsletter. And I liked that idea. Going forward, in every week’s newsletter, I’m going to include a photo I clicked just to give the newsletter a bit of a “personal touch” :)
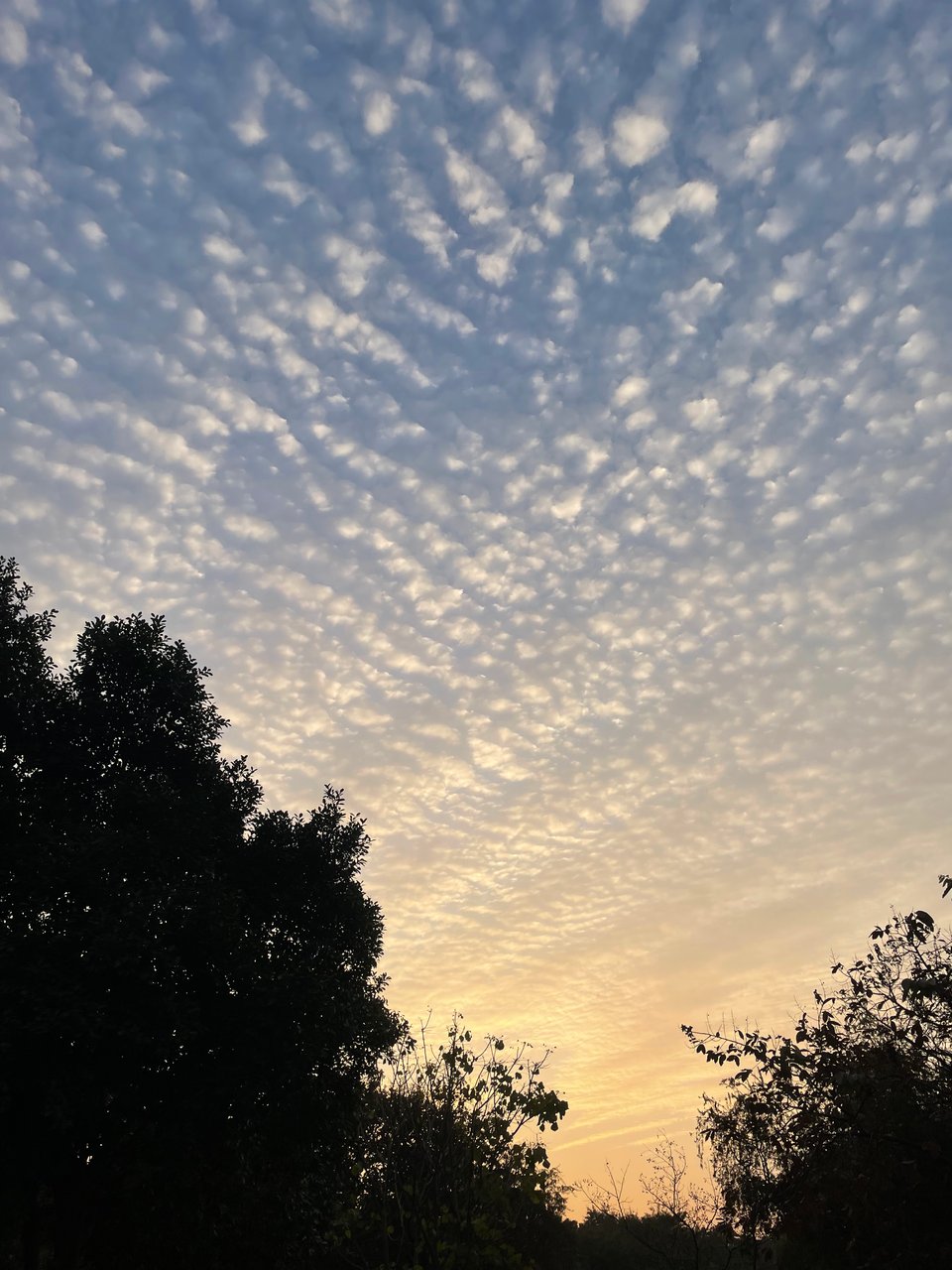
Interesting links for the week
Instead of sending a bunch of random links your way, these are all things I’ve read or seen and found interesting and relevant enough to share!
Something that sparked my curiosity
I’ve thought about the idea of starting my own YouTube channel, but I always found an excuse not to do it. I told myself I’m not an “expert” at anything, so who’d even want to watch me? Well, I realized that maybe I shouldn’t care about that. The most common advice I’ve heard when it comes to YouTube is that you need to create content that you enjoy because if you don’t, you won’t be able to stick with it. So, I thought, why not do just that and let the universe judge whether somebody wants to see it or not? Worst case, nobody sees it, but I still have fun making those videos.
So, what’s that thing I really like and would continue doing even if I get no views? Turns out, it’s learning new things. I love to experiment and try out new stuff. I’m going to start a channel that allows me to do just that. Stay tuned for it! :)
Golang puzzle of the week
Your task is to write a function CheckTicTacToe
that takes a 3x3 Tic Tac Toe board as input (represented as a 2D array) and returns the state of the game. The function should return:
"X wins"
if X has won."O wins"
if O has won."Draw"
if the game is a draw."In Progress"
if the game is still ongoing.
The board is represented as a 2D array of strings, where each cell can be "X"
, "O"
, or ""
(empty). Here’s some starter code:
package main
import (
"fmt"
)
func CheckTicTacToe(board [3][3]string) string {
// Implement your logic here
}
func main() {
// Test cases
testCases := []struct {
board [3][3]string
want string
}{
{
board: [3][3]string{
{"X", "O", "X"},
{"O", "X", "O"},
{"O", "X", "X"},
},
want: "X wins",
},
{
board: [3][3]string{
{"O", "X", "O"},
{"X", "O", "X"},
{"X", "O", "O"},
},
want: "O wins",
},
{
board: [3][3]string{
{"X", "O", "X"},
{"X", "O", "O"},
{"O", "X", "X"},
},
want: "Draw",
},
{
board: [3][3]string{
{"X", "O", "X"},
{"", "O", "O"},
{"O", "X", ""},
},
want: "In Progress",
},
{
board: [3][3]string{
{"", "", ""},
{"", "", ""},
{"", "", ""},
},
want: "In Progress",
},
{
board: [3][3]string{
{"X", "X", "X"},
{"O", "O", ""},
{"", "", ""},
},
want: "X wins",
},
{
board: [3][3]string{
{"O", "", "X"},
{"O", "X", ""},
{"O", "", "X"},
},
want: "O wins",
},
}
// Run test cases
for _, tc := range testCases {
result := CheckTicTacToe(tc.board)
fmt.Printf("Board:\\n%v\\nExpected: %s, Got: %s\\n\\n", tc.board, tc.want, result)
}
}
You can write solutions to this in the Go Playground and share the link with me on LinkedIn to get a shoutout in next week’s newsletter!
Who’s hiring?
Upbound is hiring for a Sales Development Representative across Europe (remote)
Yugabyte is hiring for a Software Engineer in India (remote)
Infisical is hiring for a Full Stack Engineer across US and Canada (remote)