“A man can only stumble for so long before he either falls or stands up straight.” - Tindwyl, The Well of Ascension
Hey hey! Hope you all had a great weekend! Mine was spent catching up with friends and reading Words of Radiance by Brandon Sanderson. Lovely series—would highly recommend if you’re into epic fantasy!
Picture of the week: This delicious Margherita pizza I had! 🍕
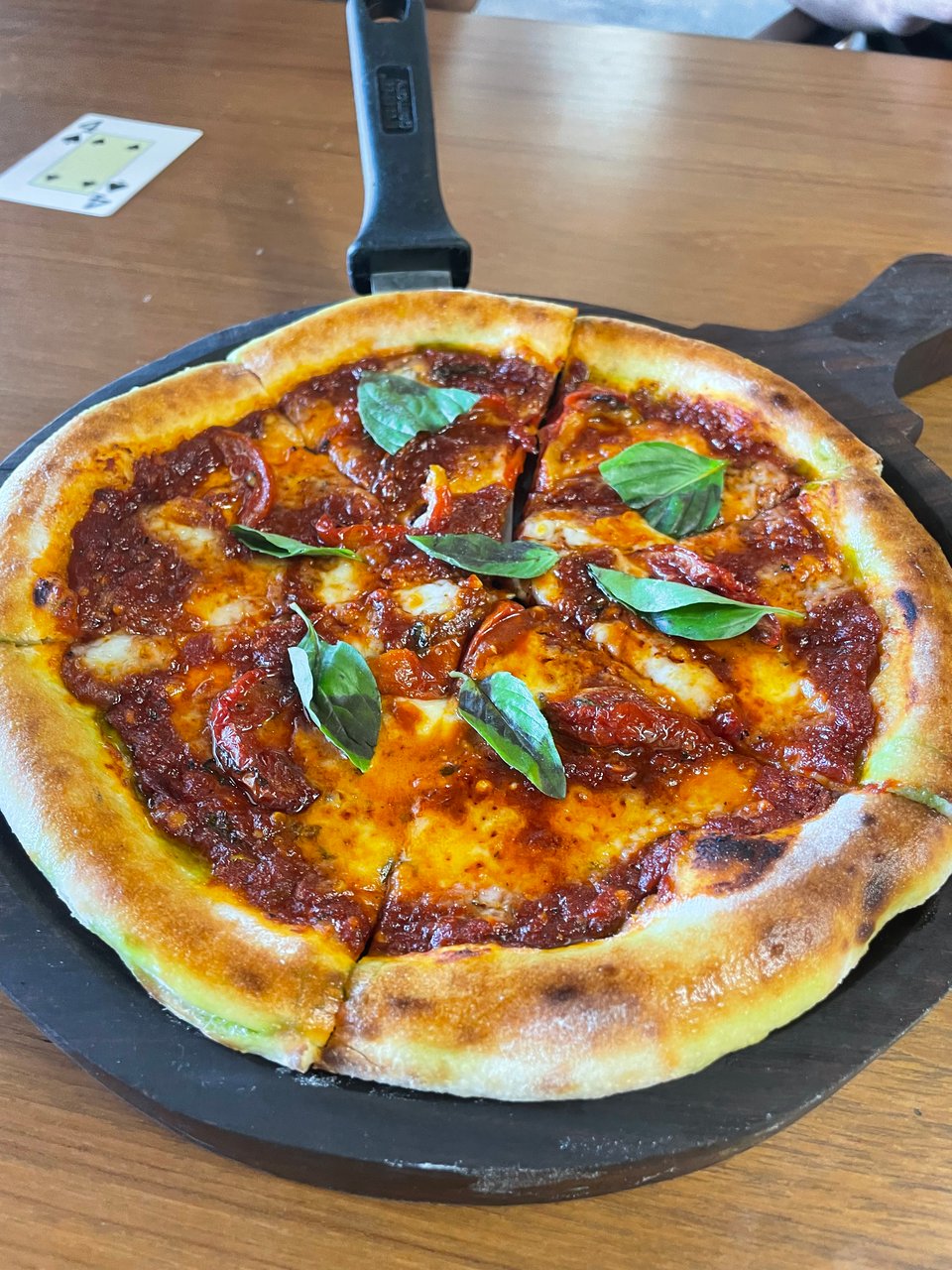
Interesting links for the week
Instead of sending a bunch of random links your way, these are all things I’ve read or seen and found interesting and relevant enough to share!
Dapr Agents: Scalable AI Workflows with LLMs, Kubernetes & Multi-Agent Coordination
Introducing k0rdent: Design, Deploy, and Manage Kubernetes-based IDPs
Something that sparked my curiosity
I’ll be at KubeCon next week, and I’m really excited to be catching up with my friends from the Kubernetes and CNCF community! If you’re attending as well, reply to this newsletter, and let’s meet up.
The last couple of KubeCons for me have been spent in platform engineering-related tracks, but this time I’m really excited about attending some AI-related talks. Expect a blog post after the conference covering my experience!
Who’s hiring?
Mirantis is hiring for a Senior Software Engineer (remote, US)
InfraCloud is hiring for a Product Engineer in India (remote)
Embrace is hiring for a Growth Marketing Specialist in US (remote)
Golang puzzle of the week
Write a function Deduplicate(nums []int) int
that removes duplicate values from a sorted integer slice in-place (without allocating a new array). The function should return the new length of the deduplicated slice.
Modify the input slice directly.
The relative order of elements must be preserved.
Assume the input is sorted in ascending order.
Space complexity must be O(1) (no extra memory except for a few variables).
Here’s some starter code:
package main
import (
"fmt"
"reflect"
)
func Deduplicate(nums []int) int {
// Your code here
}
func main() {
// Test cases
tests := []struct {
input []int
expected []int
length int
}{
{[]int{1, 1, 2}, []int{1, 2}, 2},
{[]int{1, 1, 2, 3, 3, 3, 4, 5, 5}, []int{1, 2, 3, 4, 5}, 5},
{[]int{}, []int{}, 0},
{[]int{7}, []int{7}, 1},
{[]int{0, 0, 0, 0}, []int{0}, 1},
}
for i, test := range tests {
// Create a copy of the input to preserve original for debugging
inputCopy := make([]int, len(test.input))
copy(inputCopy, test.input)
// Run the function
newLength := Deduplicate(inputCopy)
result := inputCopy[:newLength]
// Check results
if newLength != test.length || !reflect.DeepEqual(result, test.expected) {
fmt.Printf("Test %d failed:\\nInput: %v\\nGot: %v (length %d)\\nWant: %v (length %d)\\n\\n",
i+1, test.input, result, newLength, test.expected, test.length)
} else {
fmt.Printf("Test %d passed\\n", i+1)
}
}
}
You can write solutions to this in the Go Playground and share the link with me on LinkedIn to get a shoutout in next week’s newsletter!